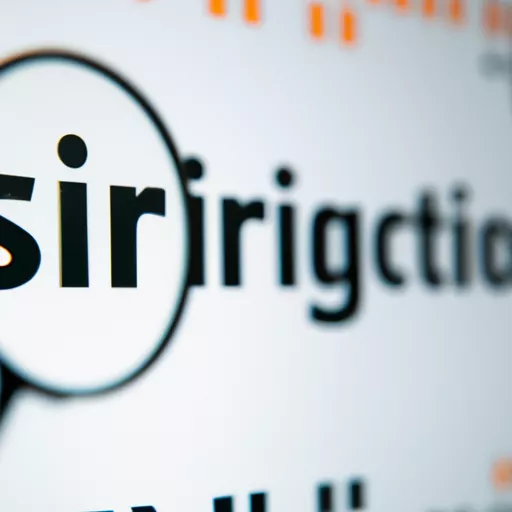
Symfony is one of the most popular and powerful frameworks for developing web applications in PHP. It provides developers with a wide range of tools and functionality that facilitate the process of creating high-quality and scalable code.
If you are a PHP developer and just starting to learn Symfony, you may be interested in where to start and what awaits you in this new world. In this article, we will look at the basics of working with Symfony and give you some tips on getting started.
Installing Symfony
The first step in mastering Symfony is installing it. There are several ways to install the framework, but one of the most popular is to use the Composer tool. Composer is a dependency manager for PHP that allows you to manage libraries and frameworks, including Symfony.
To install Symfony via Composer, you need to follow these steps:
1. Install Composer on your system following the instructions provided on the official composer.org website;
2. Create a new Symfony project using the command:
composer create-project symfony/website-skeleton my_project_name
Where "my_project_name" is the name of your new project. Composer will create a new directory and install all the necessary files and Symfony dependencies into it.
After successfully creating the project, you will have access to the basic Symfony structure and all the necessary files to start development.
Key Concepts of Symfony
Before starting to work with Symfony, it is important to understand some of its key concepts.
Controllers: Controllers in Symfony are classes that handle user requests and return the corresponding response. This is one of the core components of the framework.
Routes: Routing is the process by which a user request is directed to the appropriate controller based on the URL. In Symfony, routes are defined in the routes.yaml file and contain information about the URL and the corresponding controller.
Views: Views are responsible for displaying the data received from the controller and presenting it in a readable format. Symfony uses templating engines such as Twig to create views.
Entities: Entities in Symfony are objects that correspond to records in a database. They help manage data and provide an interface for working with the database in an object-oriented style.
Services: Services in Symfony provide various functionality that can be used in the application. They encapsulate logic and can be used in different parts of the code.
Creating a Simple Web Application
Now that you have a basic understanding of Symfony and its key concepts, you can try creating a simple web application.
As an example, let's create an application for managing tasks. First, create a controller that will handle the requests. Create a new file in the src/Controller directory and name it TaskController.php.
Inside TaskController.php, insert the following code:
namespace App\Controller;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\HttpFoundation\Response;
class TaskController extends AbstractController
{
public function index(): Response
{
return $this->render('task/index.html.twig', [
'tasks' => ['Task 1', 'Task 2', 'Task 3'],
]);
}
}
In this example, we created a TaskController class that extends AbstractController. Inside the class, we defined an index() method that returns a response in the form of a view. We pass an array of tasks to the view through the second parameter of the render() method.
Now let's create a view for displaying the tasks. In the templates/ directory, create a new directory named task, and inside it create a file named index.html.twig.
Inside index.html.twig, insert the following code:
{% extends 'base.html.twig' %}
{% block content %}
{% for task in tasks %}
<p>{{ task }}</p>
{% endfor %}
{% endblock %}
In this example, we created a base template that extends the Symfony base template. Inside the content block, we use a loop to iterate over the tasks and display them on the page.
The final step is to configure the route for our controller. Open the routes.yaml file and add the following code:
task_index:
path: /
controller: App\Controller\TaskController::index
This defines a route / that directs requests to the index() method in the TaskController.
Now you can start the Symfony server using the command:
php bin/console server:run
And navigate to http://localhost:8000 to see the result. You should see a list of tasks that we passed to the view.
Conclusion
In this article, we covered the basics of working with the Symfony framework. We learned how to install Symfony, familiarized ourselves with its key concepts, and created a simple web application. Symfony provides a wide range of tools and capabilities that help developers quickly and efficiently create web applications in PHP. If you are just starting to explore Symfony, feel free to experiment and explore new possibilities to get the most out of this powerful framework.