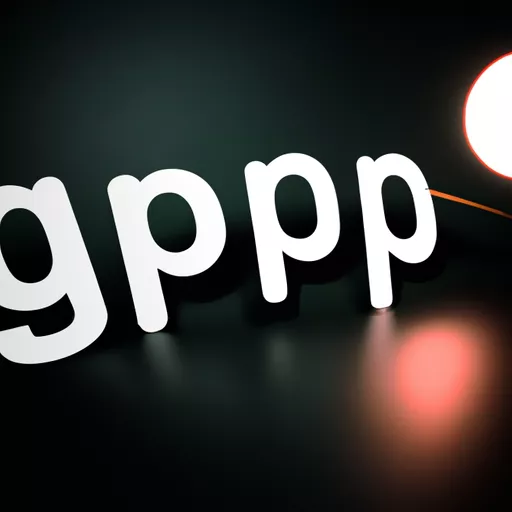
GraphQL (Graph Query Language) is a query language and server-side query execution environment for developing and maintaining client APIs. It was developed by Facebook in 2012 and first introduced to the public in 2015. GraphQL allows clients to precisely specify the structure of the data they want to receive and provides a single entry point for all queries.
One of the main goals of GraphQL is to improve the efficiency of communication between the client and server. Unlike traditional REST APIs, which often return fixed sets of data, GraphQL allows the client to request only the data it needs. This reduces the load on the server and decreases the amount of transferred data.
Creating an efficient API using GraphQL can be broken down into several steps:
Step 1: Defining the schema
The GraphQL schema defines the data types and relationships between them. It describes the structure of the data that can be obtained through the API and the actions that can be performed with this data. The GraphQL schema consists of types, fields, and directives.
Types describe the structure of data. They can be objects, scalars (strings, numbers, booleans), or enumerations. Fields define what data can be obtained and what actions can be performed. Directives allow you to control the execution of a query and the flow of data.
Example GraphQL schema:
type User { id: ID! name: String! email: String! posts: [Post!]! } type Post { id: ID! title: String! content: String! author: User! } type Query { user(id: ID!): User postsByAuthor(authorId: ID!): [Post!]! }In this example, the schema defines three data types: User, Post, and Query. The User type has fields id, name, email, and posts. The Post type has fields id, title, content, and author. The Query type has two fields: user and postsByAuthor.
Step 2: Implementing resolvers
Resolvers are functions that define how to retrieve data for a specific field. Each field in the GraphQL schema must have a corresponding resolver.
Resolvers can retrieve data from various sources, such as a database, HTTP requests, or other APIs. They can also combine data from different sources or perform complex operations with data.
Example resolver for the user field:
function getUser(id) { // retrieve user data from the database } const resolvers = { Query: { user: (parent, { id }) => getUser(id), }, User: { posts: (parent) => getPostsByAuthor(parent.id), }, }In this example, the resolver for the user field retrieves user data from the database using the getUser function. The resolver for the posts field of the User type retrieves the user's posts using the getPostsByAuthor function, which also retrieves data from the database.
Step 3: Setting up the GraphQL server
A GraphQL server is essentially a server that accepts and processes GraphQL queries. There are several popular packages for setting up a GraphQL server, such as Apollo Server, Express GraphQL, and GraphQL Yoga.
Example setup of Apollo Server:
const { ApolloServer } = require('apollo-server'); const typeDefs = require('./schema'); const resolvers = require('./resolvers'); const server = new ApolloServer({ typeDefs, resolvers, }); server.listen().then(({ url }) => { console.log(`Server started at ${url}`); });In this example, an Apollo Server is created with the provided schema (typeDefs) and resolvers. The server is then started and listens on the specified port.
Step 4: Developing the client application
After setting up the GraphQL server, you can develop a client application that will utilize this API. There are also several popular packages for client-side GraphQL development, such as Apollo Client, Relay, and Urql.
Example query using Apollo Client:
import { ApolloClient, gql, InMemoryCache } from '@apollo/client'; const client = new ApolloClient({ uri: 'http://localhost:4000', cache: new InMemoryCache(), }); const GET_USER = gql` query getUser($id: ID!) { user(id: $id) { id name email } } `; client.query({ query: GET_USER, variables: { id: '1' }, }).then((result) => { console.log(result.data.user); });In this example, the Apollo Client sends the getUser query to the server using the query method. The query has a variable id and retrieves the fields id, name, and email for the user with the specified id. The result of the query is logged to the console.
Step 5: Optimizing queries using directives
Directives are instructions for query execution or data processing on the server. They allow you to control the execution of a query and optimize it.
Example usage of the @skip directive:
const GET_USER = gql` query getUser($id: ID!, $withPosts: Boolean!) { user(id: $id) { id name email posts @skip(if: $withPosts) } } `; client.query({ query: GET_USER, variables: { id: '1', withPosts: true }, }).then((result) => { console.log(result.data.user); });In this example, the @skip directive is used to skip the posts field if the value of the withPosts variable is true. This way, you can choose whether to include the user's posts in the query.
GraphQL provides other directives as well, such as @include, @deprecated, and @auth. They allow you to flexibly control the execution of queries and provide data to clients.
GraphQL offers an efficient and flexible way to create APIs for websites and applications. With GraphQL, you can optimize the communication between the client and server, as well as improve the performance and scalability of your application. By being creative and experimenting with the various possibilities of GraphQL, you can create a powerful and efficient API for your website.