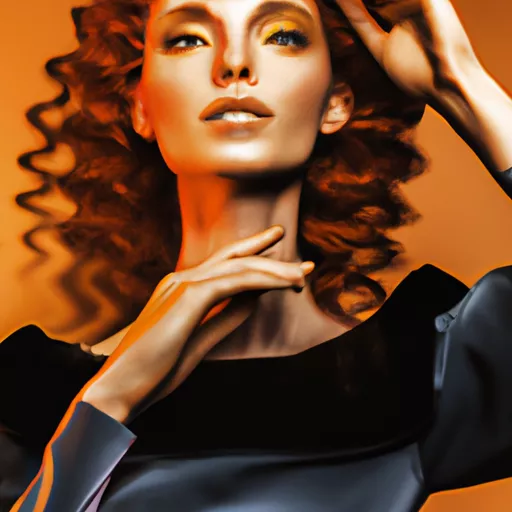
Website creation can become a complex and labor-intensive process, especially for those who are encountering it for the first time. One way to facilitate development and relieve headaches is to use module bundlers, such as Webpack. In this master class, we will cover the effective use of Webpack for creating websites and how this tool can help streamline and speed up development.
Webpack is a powerful and flexible module bundler that is designed to work with projects of any complexity. It allows you to combine and optimize various resources, such as CSS, JavaScript, HTML, and images, with minimal effort and without additional work. Here's how to do it:
First, you need to install Webpack using the command:
npm install -g webpackThen create a configuration file called webpack.config.js which will contain the settings for your project. In this file you can specify various options and plugins that will help optimize your code and reduce traffic volume. For instance:
const path = require('path'); module.exports = { entry: './src/index.js', output: { filename: 'main.js', path: path.resolve(__dirname, 'dist'), }, module: { rules: [ { test: /\.css$/, use: [ 'style-loader', 'css-loader', ], }, ], }, };Here we specified the entry point, which is in the index.js file. Then we indicated that the output should be a file called main.js, which will be saved in the /dist folder. In the rules section, we added handlers for CSS files that will be used to optimize styles.
Now you can run the build of your project using the command:
webpackWebpack will automatically process all specified resources, combine them into one file, and minify the code, which will significantly simplify development and speed up website loading.
However, plugins come to the aid, which allow you to expand the capabilities of Webpack and optimize additional resources. For example:
const HtmlWebpackPlugin = require('html-webpack-plugin'); module.exports = { plugins: [ new HtmlWebpackPlugin({ template: 'src/index.html', }), ], };In this example, we added the HtmlWebpackPlugin plugin, which will automatically insert the generated JavaScript code into the specified HTML template. Thus, you don't need to manually add a link to the compiled script in your HTML file.
Another useful plugin is the MiniCssExtractPlugin, which allows you to extract all CSS styles into a separate file, improving performance and simplifying development:
const MiniCssExtractPlugin = require('mini-css-extract-plugin'); module.exports = { plugins: [ new MiniCssExtractPlugin({ filename: '[name].css', }), ], module: { rules: [ { test: /\.css$/, use: [ MiniCssExtractPlugin.loader, 'css-loader', ], }, ], }, };By using module bundlers such as Webpack, you can significantly simplify and speed up the website development process. You no longer have to manually manage all resources and embed links in HTML code, as Webpack takes care of this automatically. Thanks to the use of plugins and the presence of numerous options, this tool will become an indispensable assistant in creating high-quality and fast websites without headaches.