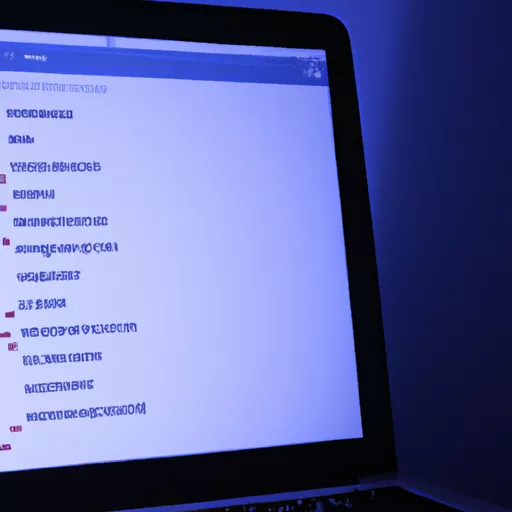
Node.js is a JavaScript runtime environment that allows you to run JavaScript code on the server. The main feature of Node.js is its asynchronous execution model, which allows it to handle a large number of requests simultaneously, making it highly efficient for building server applications.
To get started with Node.js, you will need to install it. You can download the Node.js installer from the official website and run it on your computer. After a successful installation, you can open the command prompt and check the installed Node.js version using the command node -v.
Once Node.js is successfully installed, you can proceed to create a server. To do this, you need to create a new file with the .js extension and add the following code:
```javascript const http = require('http'); const server = http.createServer((request, response) => { response.writeHead(200, {'Content-Type': 'text/plain'}); response.end('Hello, World!\n'); }); server.listen(8080, 'localhost', () => { console.log('Server running at http://localhost:8080/'); }); ```In this example, we used the http module to create a simple HTTP server. We defined an event handler createServer, which will be called for each new request. Inside the handler, we use the methods of the response object to set response headers and send data to the client.
To run this server, save the file with the .js extension and execute it using the command node server.js. Now your server will be up and running, responding to requests at http://localhost:8080/.
Node.js also provides a set of modules for working with databases. For example, you can use the mongoose module to connect to and interact with MongoDB, one of the most popular NoSQL databases.
To use the mongoose module, you need to install it using the command npm install mongoose. After installation, you can require it in your file using the command const mongoose = require('mongoose');
To connect to a database, you should use the mongoose.connect() method, passing it the database URL and connection options. For example:
mongoose.connect('mongodb://localhost/mydatabase', { useNewUrlParser: true, useUnifiedTopology: true });After successfully connecting to the database, you can use mongoose methods to create, read, update, and delete data. For example:
// Creating a new document const user = new User({ name: 'John Doe', age: 25, email: 'johndoe@example.com' }); await user.save(); // Reading data const users = await User.find(); console.log(users); // Updating data user.age = 26; await user.save(); // Deleting data await user.remove();In this example, we used a User model created using the mongoose.model() method. A model represents a collection of documents in the database and allows us to use mongoose methods to work with data.
Node.js is great for building server applications, thanks to its asynchronous execution model and powerful modules available in its ecosystem. I hope this brief overview helps you get started with Node.js and server-side development.